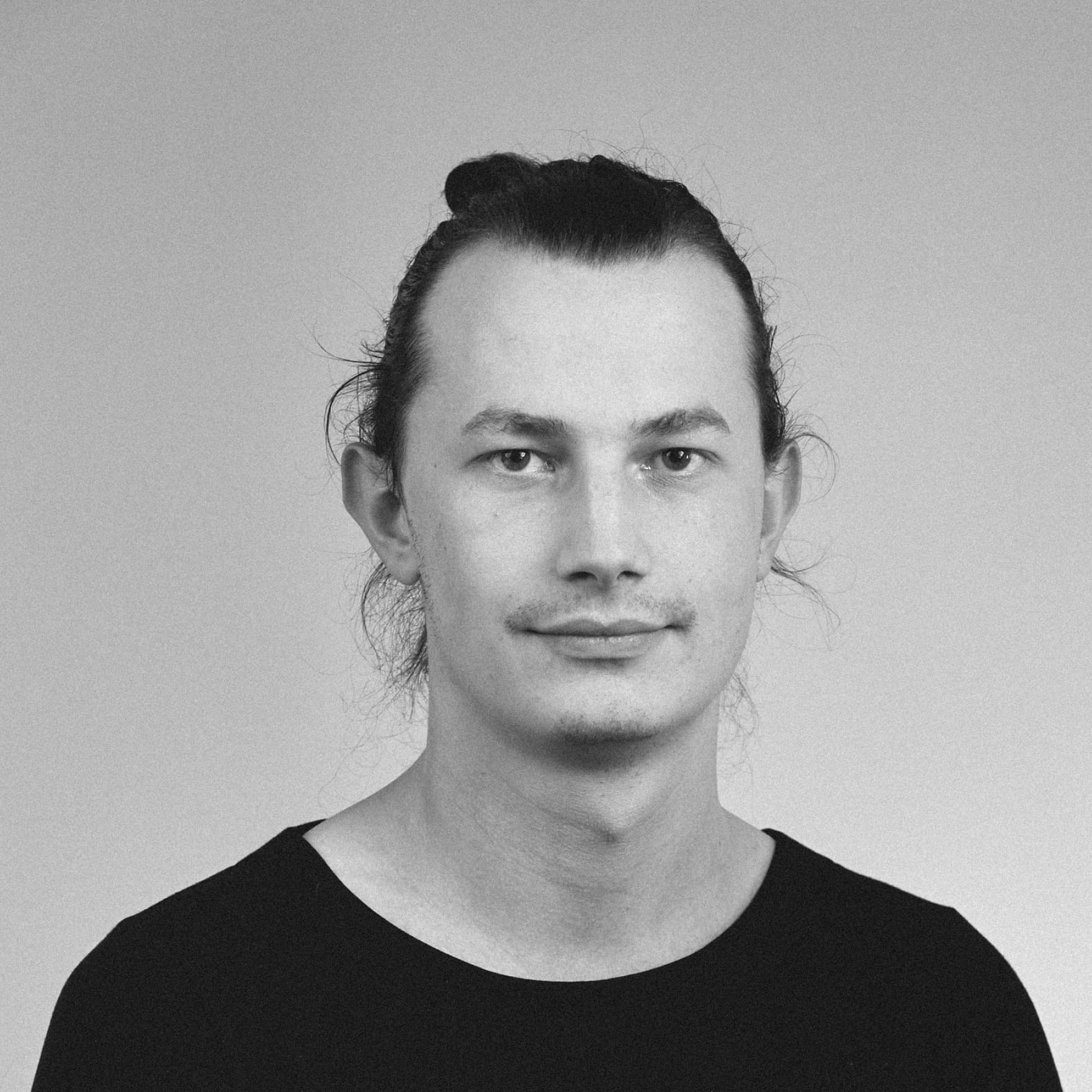
In modern application development, using S3 for file storage has become increasingly common. For many applications, files represent a core aspect of the domain. S3 provides a simple and reliable solution for storing and retrieving files. However, testing S3 integration is not always straightforward. In this blog post, we will explore practical strategies for testing S3 integrations in your integration tests.
Common Approaches for Testing S3 Integrations
Two main approaches are widely adopted when it comes to testing S3 calls in integration tests. The first, and perhaps the simplest, is the use of mocks. By mocking the S3 calls, you can verify that the expected actions are triggered without making real network calls. While this approach is relatively easy to implement, it has significant drawbacks:
- Manual Setup: You have to handle every aspect of the test yourself. This includes managing the mock environment, setting up file uploads and downloads, and configuring the test data for each scenario.
- Lack of Realism: Mocking only verifies that the calls are made, but it doesn't test the actual interaction with S3, which could lead to discrepancies between the mock and the system's actual behavior.
Although utility classes can be created to streamline some of these tasks, this approach remains somewhat cumbersome and lacks the real-world fidelity you may need for more thorough tests.
A more practical alternative is LocalStack. This open-source tool creates a fully functional local environment for AWS cloud services, notably S3. It enables you to emulate AWS services on your local machine without requiring an actual AWS account or making real network calls.
The advantage of LocalStack is that it closely mirrors the behavior of the actual AWS cloud, making your tests more reliable and realistic. You can perform tests that interact with a local instance of S3, just as with AWS.
However, LocalStack also presents some challenges:
- Complex Setup: LocalStack emulates a wide range of AWS services, making it difficult to configure correctly. Depending on your application, you may need to account for interactions with other AWS services (e.g., SQS, SNS, DynamoDB) as part of your test setup.
- Performance Considerations: While LocalStack is powerful, it can be resource-intensive, especially if you are emulating a large number of services.
- Cost: Although LocalStack offers an open-source version, it does not include all features. You may need to purchase a commercial license to access premium functionalities, such as support for additional AWS services and advanced features like parallel testing.
Both approaches come with trade-offs, and the choice largely depends on the specific needs of your project.
Using Testcontainers with MinIO
The final approach we’ll explore is leveraging Testcontainers, a Java library that simplifies managing and interacting with Docker containers in tests. With Testcontainers, you can effortlessly spin up fully configured, disposable containers for services like S3-compatible storage. A popular example of such storage is MinIO, a high-performance, self-hosted object storage solution fully compatible with the Amazon S3 API.
This approach offers significant convenience, enabling you to run an S3-compatible container with minimal setup—often with just a single annotation on the test class.
Let’s take a closer look at how this works in practice. To start a MinIO container in your tests, you only need to add the following lines at the beginning of your test class:
1private static final MinIOContainer CONTAINER = new MinIOContainer("minio/minio")
2 .withUserName("testuser")
3 .withPassword("testpassword");
4
5@BeforeAll
6public static void startServer() throws IOException {
7 CONTAINER.start();
8}
9
With this setup, Testcontainers will automatically pull the MinIO image (if it’s not already available locally) and start a MinIO container before your tests begin. Once all tests in the class are complete, the container is destroyed and cleaned up.
While this is incredibly convenient, one limitation is that you might find it cumbersome to add this snippet to every test class. This is where a useful feature of JUnit comes into play.
JUnit Extensions
JUnit provides a powerful feature that allows you to create custom extensions, enabling you to extend the framework's functionality in a modular and reusable way. Using JUnit extensions, we can encapsulate our container setup into a separate class and reuse it across multiple test classes, simplifying our test configuration.
Here’s an example of how you might implement such an extension for MinIO:
1public class MinIOTestcontainers implements BeforeAllCallback {
2 private static final MinIOContainer CONTAINER = new MinIOContainer("minio/minio")
3 .withUserName("testuser")
4 .withPassword("testpassword")
5 .withReuse(true);
6
7 @Override
8 public void beforeAll(ExtensionContext context) {
9 CONTAINER.start();
10
11 Integer mappedPort = CONTAINER.getFirstMappedPort();
12 String url = String.format("http://%s:%s", CONTAINER.getHost(), mappedPort);
13 System.setProperty("s3.endpoint", url);
14 }
15}
16
In this implementation, we define our MinIO container just as we did previously. The key difference is that we implement the BeforeAllCallback
interface from JUnit, which allows us to hook into the beforeAll
lifecycle method. In this method, we start the container and set the s3.endpoint
property, which, in this case, configures the connection to MinIO.
One important enhancement here is the use of .withReuse(true)
. This option tells Testcontainers to reuse the same container across all test classes, instead of starting a new container for each class. This dramatically reduces execution time, especially for large test suites, by avoiding the overhead of repeatedly starting and stopping containers.
Once the extension is defined, all we need to do is annotate any test class that requires an S3 bucket with @ExtendWith(MinIOTestcontainers.class)
, like this:
1@ExtendWith(MinIOTestcontainers.class)
2public class YourTestClass {
3 // Test methods here
4}
5
This makes it extremely easy to reuse the container setup across multiple test classes, keeping the test code clean and consistent.
Setting and Cleaning Up Buckets in Integration Tests
So far, we have focused on starting and connecting to the MinIO server. However, we have not yet addressed bucket creation and cleanup. MinIO does not create buckets by default, so we must manually create them before running tests. Additionally, to ensure test isolation and avoid issues with pre-existing buckets or leftover data, we should clean up any created buckets after each test.
One way to handle this is to manually create a bucket at the beginning of each test class and delete it at the end of each test. However, this approach introduces unnecessary boilerplate code. Instead, we can extend our MinIOTestcontainers
JUnit extension to handle bucket management automatically.
Extending the JUnit Extension for Bucket Cleanup
To ensure proper bucket cleanup after each test, we begin by initializing a MinIO client using the beforeAll
method. This establishes a reliable connection to MinIO for all tests. Then, we implement AfterEachCallback
to automatically delete all buckets and their contents after each test, preventing data conflicts and ensuring each test runs in an isolated environment.
Here’s the updated implementation:
1public class MinIOTestcontainers implements BeforeAllCallback, AfterEachCallback {
2 private static final MinIOContainer CONTAINER = new MinIOContainer("minio/minio")
3 .withUserName("testuser")
4 .withPassword("testpassword")
5 .withReuse(true);
6
7 private static MinioClient minioClient;
8
9 @Override
10 public void beforeAll(ExtensionContext context) {
11 CONTAINER.start();
12
13 Integer mappedPort = CONTAINER.getFirstMappedPort();
14 String url = String.format("http://%s:%s", CONTAINER.getHost(), mappedPort);
15 System.setProperty("s3.endpoint", url);
16
17 try {
18 minioClient = MinioClient.builder()
19 .endpoint(url)
20 .credentials("testuser", "testpassword")
21 .build();
22 } catch (Exception e) {
23 throw new RuntimeException("Failed to initialize MinIO client", e);
24 }
25 }
26
27 @Override
28 public void afterEach(ExtensionContext context) {
29 try {
30 for (Bucket bucket : minioClient.listBuckets()) {
31 var items = minioClient.listObjects(ListObjectsArgs.builder().bucket(bucket.name()).recursive(true).build());
32 for (var item : items) {
33 minioClient.removeObject(RemoveObjectArgs.builder()
34 .bucket(bucket.name())
35 .object(item.get().objectName())
36 .build());
37 }
38 minioClient.removeBucket(RemoveBucketArgs.builder().bucket(bucket.name()).build());
39 }
40 } catch (Exception e) {
41 throw new RuntimeException("Failed to clean up MinIO buckets", e);
42 }
43 }
44}
45
By automating cleanup, we eliminate manual intervention and ensure a consistent test environment, making integration tests more reliable and efficient.
Automating Bucket Creation
To streamline the test setup even further, we can implement BeforeEachCallback
to automatically create a test bucket before each test. This ensures a fresh bucket is available for every test while maintaining proper test isolation.
We also optimized the afterEach
cleanup method by limiting it to only delete the test bucket, instead of removing all buckets. This helps prevent unintended deletions should additional buckets exist in the test container.
Here’s how to integrate the automated bucket creation:
1public class MinIOTestcontainers implements BeforeAllCallback, BeforeEachCallback, AfterEachCallback {
2 private static final MinIOContainer CONTAINER = new MinIOContainer("minio/minio")
3 .withUserName("testuser")
4 .withPassword("testpassword")
5 .withReuse(true);
6
7 private static MinioClient minioClient;
8 private static final String TEST_BUCKET_NAME = "test-bucket";
9
10 @Override
11 public void beforeAll(ExtensionContext context) {
12 // Same as before
13 }
14
15 @Override
16 public void beforeEach(ExtensionContext context) {
17 try {
18 boolean bucketExists = minioClient.bucketExists(BucketExistsArgs.builder().bucket(TEST_BUCKET_NAME).build());
19 if (!bucketExists) {
20 minioClient.makeBucket(MakeBucketArgs.builder().bucket(TEST_BUCKET_NAME).build());
21 }
22 } catch (Exception e) {
23 throw new RuntimeException("Failed to create test bucket", e);
24 }
25 }
26
27 @Override
28 public void afterEach(ExtensionContext context) {
29 try {
30 boolean bucketExists = minioClient.bucketExists(BucketExistsArgs.builder().bucket(TEST_BUCKET_NAME).build());
31 if (bucketExists) {
32 var items = minioClient.listObjects(ListObjectsArgs.builder().bucket(TEST_BUCKET_NAME).recursive(true).build());
33 for (var item : items) {
34 minioClient.removeObject(RemoveObjectArgs.builder()
35 .bucket(TEST_BUCKET_NAME)
36 .object(item.get().objectName())
37 .build());
38 }
39 minioClient.removeBucket(RemoveBucketArgs.builder().bucket(TEST_BUCKET_NAME).build());
40 }
41 } catch (Exception e) {
42 throw new RuntimeException("Failed to clean up the test bucket", e);
43 }
44 }
45}
46
With this approach:
- If a new test bucket doesn't already exist, it is automatically created before each test, eliminating the need for manual bucket creation.
- The test bucket is cleaned up after each test, ensuring that there are no conflicts between tests and that the environment remains consistent.
- MinIO container reuse optimizes test execution by reducing overhead, as the same container is used across multiple test classes.
By implementing these enhancements, we eliminate repetitive setup code in our test classes while improving test reliability and maintainability.
Room for Improvements
The approach outlined in this blog post provides a solid foundation for writing integration tests with MinIO and Testcontainers. However, there is still plenty of room for improvement.
For example, the JUnit extension could be further enhanced with:
- Dynamic bucket creation to allow tests to specify bucket names at runtime.
- Performance optimizations such as caching mechanisms or more efficient cleanup strategies.
- Additional abstractions to simplify test setup and reduce boilerplate even further.
These are topics for another time, but the concepts covered here should give you a strong starting point to build reliable and maintainable integration tests. If you have any questions or feedback, feel free to reach out to us at info@aboutbits.it